- Visual Basic 6.0 Code For Simple Calculator
- Visual Basic Simple Calculator Code Pdf
- Visual Basic Code For Simple Calculator Program
VB.NET GUI
Basic Calculator
In the previous sections, we displayed text in console window (black background window). In this section, you will learn to use window forms and other useful components and controls to create GUI applications that increase interactivity.
- In this tutorial, I would like to share you this project called Calculator in Visual Basic. This project support the basic functions of addition, subtraction, division and multiplication, as well as changing the sign of the number the '+/-' key. It's up to you if you want to support the sqrt(√), percent(%), and (1/x) functions.
- Python Program to Make a Simple Calculator In this example you will learn to create a simple calculator that can add, subtract, multiply or divide depending upon the input from the user. To understand this example, you should have the knowledge of the following Python programming topics.
Why is it simple calculator? It has only the basic functions of a calculator such as addition, subtraction, multiplication,division and 2 others. First Entered number is taken to the first variable a. Then select and operator, enter the second number. Since, Visual Basic.Net provides features where you can create quickapplication in a minute. In this tutorial, I’ll show you how to create simple calculator in Visual Basic.Net. Unfortunately, it only covers very simple calculation with four arithmetic operators such as Addition, Subtraction, Multiplication, and Division. In this example, you build a very simple calculator named SimpleCalculator. The goal of SimpleCalculator is to create a console application that accepts basic arithmetic commands, in the form '5+3' or '6-2', and returns the correct answers. Using MEF, you will be able to add new operators without changing the application code.
Step1: Create a project (Windows Forms Application)

Step2: Design interface
-Click on Toolbox in the left site to extend it. You will a list of controls as shown in below:
Visual Basic 6.0 Code For Simple Calculator
-Drag and drop one textbox and rename it to txtbox(change text in Name field of the properties window to txtbox).
Note: To open Properties window of a control, right-click the control and click Properties.
-Drag and drop 21 command buttons
-Rename button0 to cmd0, button1 to cmd1, button2 to cmd2, button3 to cmd3, button4 to cmd4, button5 to cmd5, button6 to cmd6, button7 to cmd7, button8 to cmd8, button9 to cmd9, button10 to cmdequal, button11 to cmdclear, button 12 to cmdadd, button13 to cmdsubtract, button14 to cmdmultiply, button15 to cmddivide, button16 to cmdsquare, button17 to cmdsqtr, button 18 to cmdcos, button19 to cmd sin, button20 to cmdtan.
-The caption of each button also needs to be changed. For example, you need to change the caption of button0 to 0, button1 to 1, button 2 to 2...etc.
Step3: Write code
To attach code to a control, you need to double-click the control to open code editor. And then you just copy code and paste it.
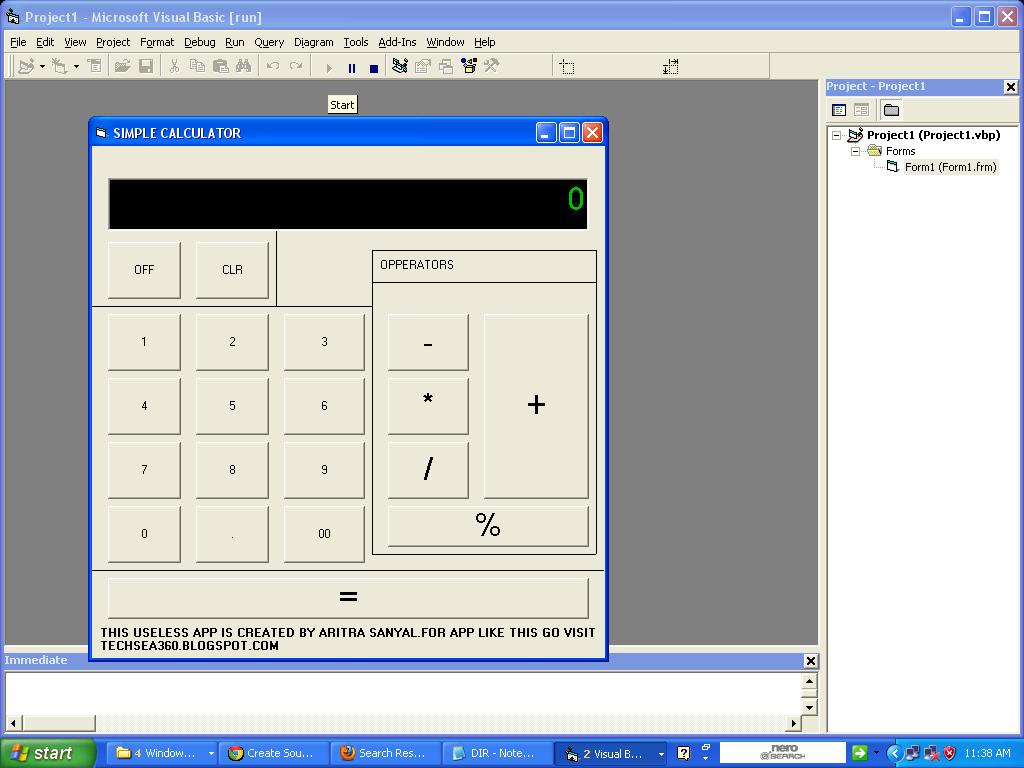
'Declaring global variables in General section of the form
Dim sign As String
Dim val1 As Double
Dim val2 As Double
Private Sub cmd0_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd0.Click
txtbox.Text = txtbox.Text & cmd0.Caption 'get 0
End Sub
Private Sub cmd1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd1.Click
txtbox.Text = txtbox.Text & cmd1.Caption 'get 1
End Sub
Private Sub cmd2_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd2.Click
txtbox.Text = txtbox.Text & cmd2.Caption 'get 2
End Sub
Private Sub cmd3_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd3.Click
txtbox.Text = txtbox.Text & cmd3.Caption 'get 3
End Sub
Private Sub cmd4_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd4.Click
txtbox.Text = txtbox.Text & cmd4.Caption 'get 4
End Sub
Private Sub cmd5_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd5.Click
txtbox.Text = txtbox.Text & cmd5.Caption 'get 5
End Sub
Private Sub cmd6_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handle cmd6.Click
txtbox.Text = txtbox.Text & cmd6.Caption 'get 6
End Sub
Private Sub cmd7_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd7.Click
txtbox.Text = txtbox.Text & cmd7.Caption 'get 7
End Sub
Private Sub cmd8_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd8.Click
txtbox.Text = txtbox.Text & cmd8.Caption 'get 8
End Sub
Private Sub cmd9_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmd9.Click
txtbox.Text = txtbox.Text & cmd9.Caption 'get 9
End Sub
Private Sub cmdclear_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdclear.Click
'clear Texts
txtbox.Text = '
val1 = 0
val2 = 0
sign = '
End Sub
Private Sub cmdcos_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdcos.Click
Dim v As Double
On Error GoTo aa
v = CDbl(txtbox.Text)
txtbox.Text = Math.Cos(v)
aa: Exit Sub
End Sub
Private Sub cmddivide_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmddivide.Click
sign = '/'
On Error GoTo aa
val1 = CDbl(txtbox.Text)
txtbox.Text = '
aa: Exit Sub
End Sub
Private Sub cmdequal_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdequal.Click
On Error GoTo aa
val2 = CDbl(txtbox.Text)
If (sign = '+') Then
txtbox.Text = val1 + val2
ElseIf (sign = '-') Then
txtbox.Text = val1 - val2
ElseIf (sign = '*') Then
txtbox.Text = val1 * val2
Else: txtbox.Text = val1 / val2
End If
aa: Exit Sub
End Sub
Private Sub cmdmultiply_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdmultiply.Click
sign = '*'
On Error GoTo aa
val1 = CDbl(txtbox.Text)
txtbox.Text = '
aa: Exit Sub
End Sub
Private Sub cmdplus_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdplus.Click
sign = '+'
On Error GoTo aa
val1 = CDbl(txtbox.Text)
txtbox.Text = '
aa: Exit Sub
End Sub
Private Sub cmdsin_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdsin.Click
Dim v As Double
On Error GoTo aa
v = CDbl(txtbox.Text)
txtbox.Text = Math.Sin(v)
aa: Exit Sub
End Sub
Private Sub cmdsqare_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdsquare.Click
Dim v As Double
On Error GoTo aa
v = CDbl(txtbox.Text)
txtbox.Text = v ^ 2
aa: Exit Sub
End Sub
Private Sub cmdsqrt_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdsqrt.Click
Dim v As Double
On Error GoTo aa
v = CDbl(txtbox.Text)
txtbox.Text = Math.Sqr(v)
aa: Exit Sub
End Sub
Private Sub cmdsubtract_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdsubtract.Click
sign = '-'
On Error GoTo aa
val1 = CDbl(txtbox.Text)
txtbox.Text = '
aa: Exit Sub
End Sub
Private Sub cmdtan_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles cmdtan.Click
Dim v As Double
On Error GoTo aa
v = CDbl(txtbox.Text)
txtbox.Text = Math.Tan(v)
aa: Exit Sub
End Sub
Private Sub txtbox_KeyPress(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles txtbox.KeyPress
'Allow only number, dot, and backspace characters
If Asc(e.KeyChar) >= Asc('0') And Asc(e.KeyChar) <= Asc('9') Or Asc(e.KeyChar) = 8 Or Asc(e.KeyChar) = 46 Then
Exit Sub
Else
Visual Basic Simple Calculator Code Pdf
e.KeyChar = '
End If
End Sub
Comments
Visual Basic Code For Simple Calculator Program
Akshay I have one problem, 2015-10-01 |
gowdhami sree this pgm is very useful 2015-08-11 |
david j A tutorial clearly explained showing beginning OO relationships in Forms 2015-01-19 |
nansa thank u soo much.it's really help me 2014-07-31 |
Larry Hello friends.........I have also searched for good tutorial on making calculator. 2014-02-22 |